You want to use Owncast on RK35** system-on-a-chip, but don't know how to compile ffmpeg (like me~)? In this guide I'll try to explain what to install and how to modify Owncast to detect & use hardware acceleration.
- Compile ffmpeg and install RGA and MPP drivers:
Use this guide, it's simple. You can delete some flags, but these flags are important:
--enable-gnutls
--enable-libx264
--enable-nonfree
--enable-rkmpp
--enable-version3
--enable-libdrm
- Clone Owncast repository.
git clone https://github.com/owncast/owncast
- Modify file codecs.go in /owncast/core/transcoder folder by adding following lines:
// RkmppCodec represents an instance of the Rkmpp codec.
type RkmppCodec struct{}
// Name returns the codec name.
func (c *RkmppCodec) Name() string {
return "h264_rkmpp"
}
// DisplayName returns the human readable name of the codec.
func (c *RkmppCodec) DisplayName() string {
return "Rkmpp"
}
// GlobalFlags are the global flags used with this codec in the transcoder.
func (c *RkmppCodec) GlobalFlags() string {
flags := []string{
"-hwaccel", "rkmpp",
"-hwaccel_device", "/dev/dri/renderD128",
"-c:v", "h264_rkmpp",
}
return strings.Join(flags, " ")
}
// PixelFormat is the pixel format required for this codec.
func (c *RkmppCodec) PixelFormat() string {
return "yuv420p"
}
// Scaler is the scaler used for resizing the video in the transcoder.
func (c *RkmppCodec) Scaler() string {
return ""
}
// ExtraFilters are the extra filters required for this codec in the transcoder.
func (c *RkmppCodec) ExtraFilters() string {
return ""
}
// ExtraArguments are the extra arguments used with this codec in the transcoder.
func (c *RkmppCodec) ExtraArguments() string {
return ""
}
// VariantFlags returns a string representing a single variant processed by this codec.
func (c *RkmppCodec) VariantFlags(v *HLSVariant) string {
return "";
}
// GetPresetForLevel returns the string preset for this codec given an integer level.
func (c *RkmppCodec) GetPresetForLevel(l int) string {
presetMapping := map[int]string{
0: "ultrafast",
1: "superfast",
2: "veryfast",
3: "faster",
4: "fast",
}
preset, ok := presetMapping[l]
if !ok {
defaultPreset := presetMapping[1]
log.Errorf("Invalid level for rkmpp preset %d, defaulting to %s", l, defaultPreset)
return defaultPreset
}
return preset
}
You also have to modify GetCodecs function by changing line with
if strings.Contains(line, "H.246")
to
if strings.Contains(line, "h264")
for RKMPP to show correctly in list of codecs in Owncast app.
Also modify Codec function at the very end of the file by adding
case (&RkmppCodec{}).Name():
return &RkmppCodec{}
in a case block and add
(&RkmppCodec{}).Name(): "h264_rkmpp",
in a supportedCodecs map.
That should be it.
- Run go build main.go (make sure that you have Go installed)
- Run ./owncast, go to Admin panel -> Configuration -> Server Setup and make sure you have ffmpeg binary you compiled before.
You also have to go to Configuraion -> Video -> Video Codec and select "h246_rkmpp".
I am getting a lot of dropped frames when I resize input from pretty much anything (from 1920x1200 to 1920x1080). Two or more quality presets leads to dropped frames too.
But it works!
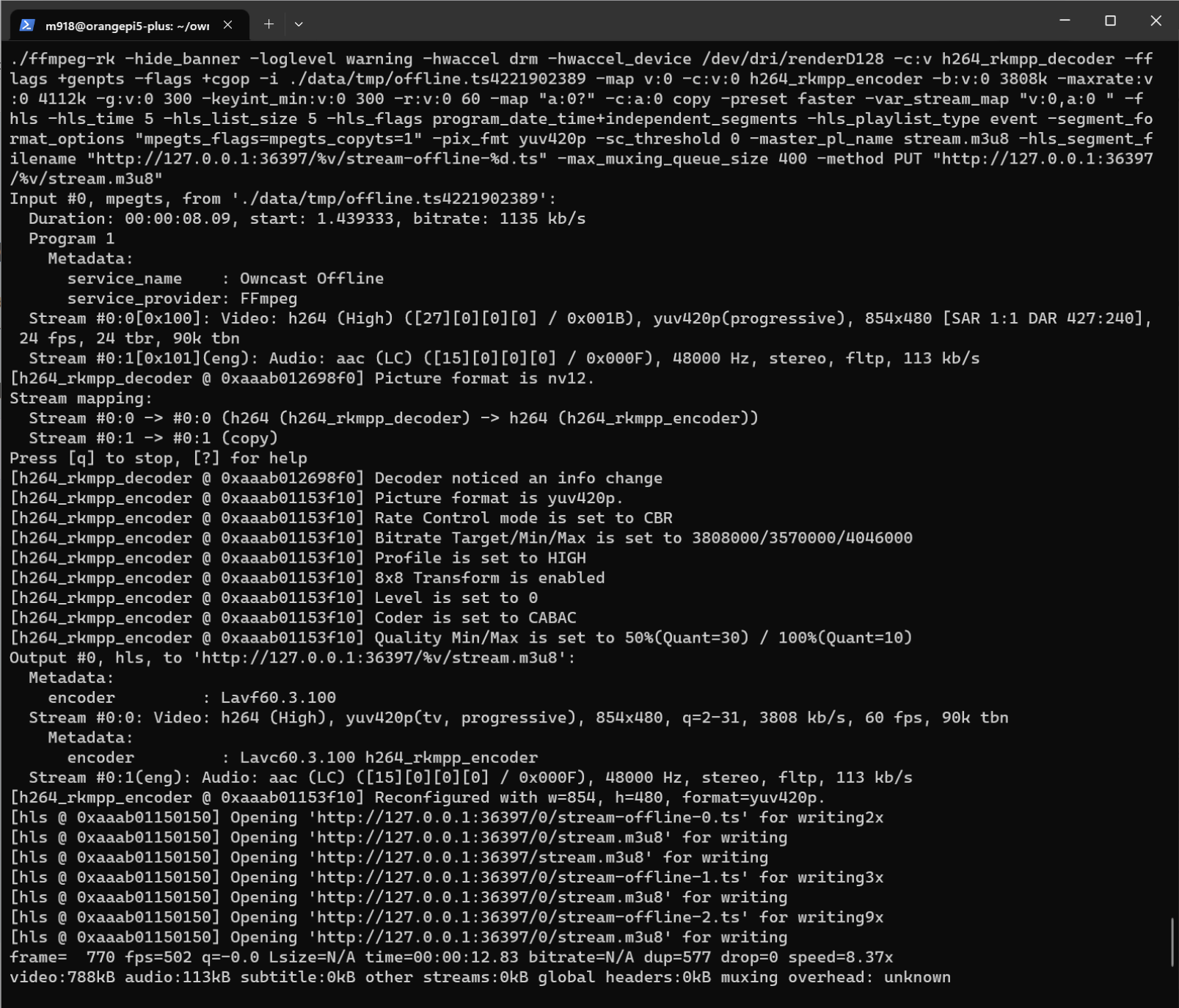
A quick note: I ran this on Armbian. Ubuntu version would work a lot better because of multimedia drivers (see this)
sudo add-apt-repository ppa:liujianfeng1994/panfork-mesa
sudo add-apt-repository ppa:liujianfeng1994/rockchip-multimedia
sudo apt update
sudo apt dist-upgrade
sudo apt install mali-g610-firmware rockchip-multimedia-config